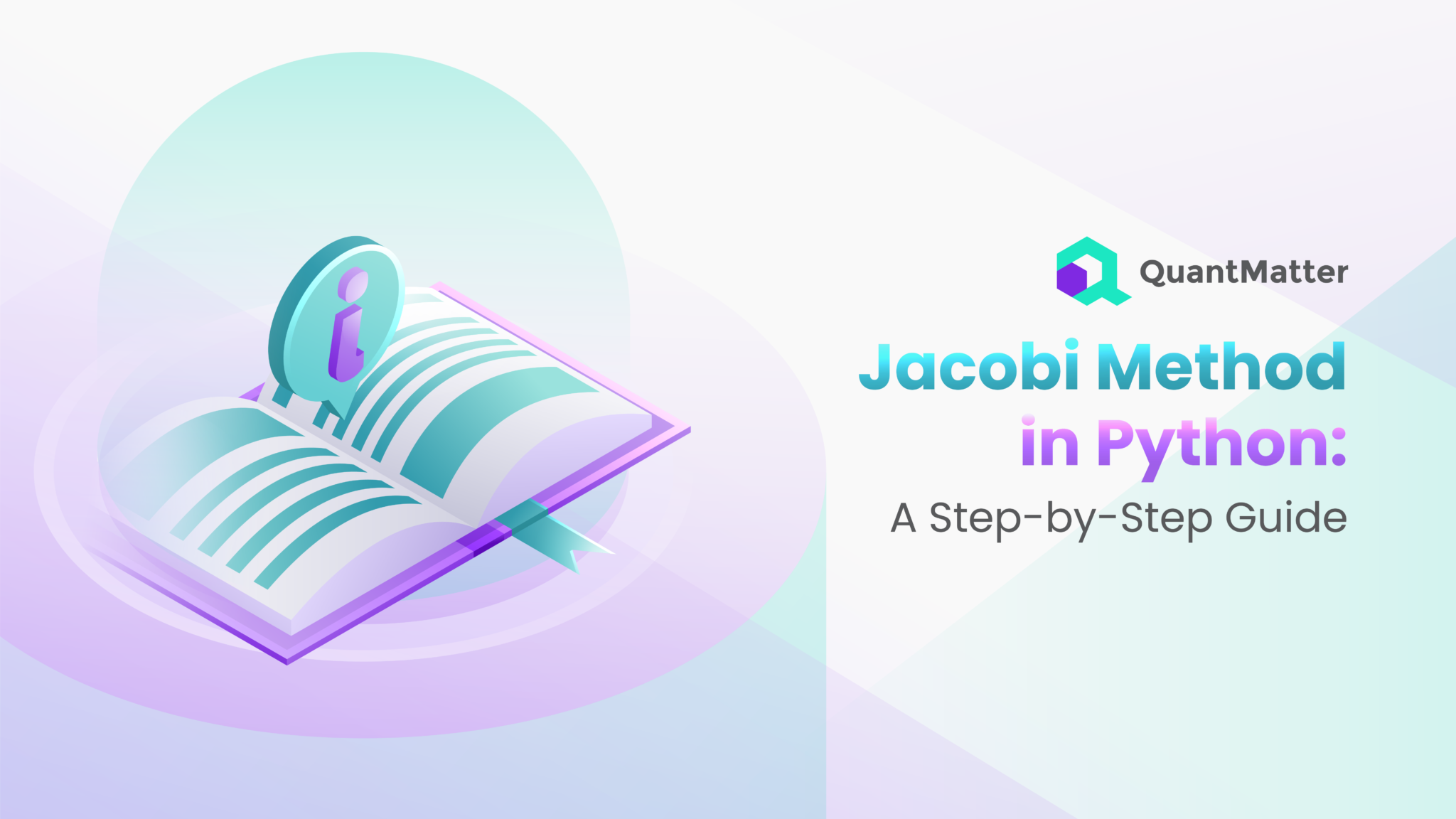
Have you ever needed to solve a bunch of equations at once? That’s what the Jacobi Method is all about. Named after the mathematician Carl Jacobi, this method gives us a way to handle systems of linear equations – those with multiple variables and equations to solve. It’s a handy technique, especially if you’re working with large sets of equations, and it’s widely used in scientific computing. If you’re a Python enthusiast, learning the Jacobi Method can open up new ways to approach math problems in code.
Now, you might be wondering, why use an iterative method like Jacobi instead of a straightforward, “solve-it-once” method? Well, the Jacobi Method doesn’t just tackle the problem in one step; instead, it breaks it down and tackles it in rounds, refining the solution each time. This makes it especially useful for large-scale problems where direct methods could be too slow or even impractical. Plus, Jacobi’s approach is naturally suited for running on multiple processors at once, which can make the solution even faster.
Using Python to code the Jacobi Method has its perks. Python is popular for scientific programming because of its readability and the power of its libraries like NumPy, which makes math operations fast and efficient. In this guide, we’ll explore the Jacobi Method from the ground up: what it is, why it’s useful, and how you can bring it to life in Python. Whether you’re solving equations for a project or just love math, let’s dive in and see what makes this method tick!
What is the Jacobi Method?
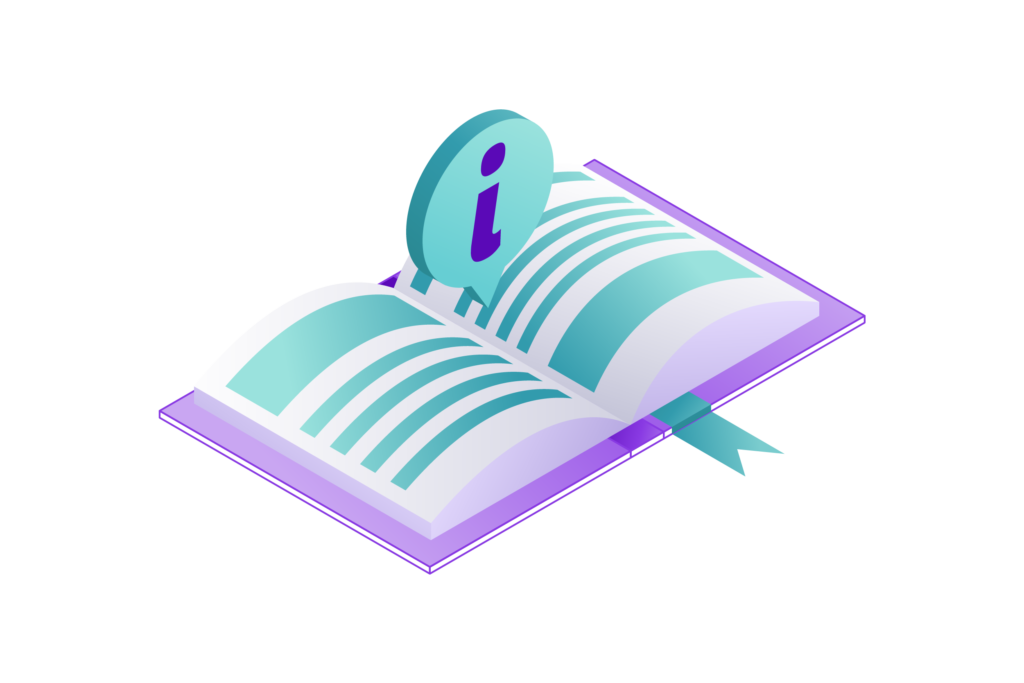
The Jacobi Method is an approach for solving systems of linear equations – essentially, a group of equations where each one includes multiple variables. Imagine you have several unknowns to figure out, and each equation gives a clue. The Jacobi Method takes all those clues and uses them to make educated guesses about the unknowns, refining those guesses step by step.
At its core, the Jacobi Method is an iterative process. This just means it doesn’t aim to get the right answer immediately. Instead, it starts with an initial guess, checks how close that guess is, and makes adjustments. This cycle of adjusting and checking continues until it gets close enough to a solution. Think of it as solving a puzzle where each piece you place gives you a better idea of where the next one goes.
One of the Jacobi Method’s biggest advantages is its ability to handle large, sparse matrices (matrices with many zeroes) efficiently. In cases like this, it’s often more practical than direct methods that attempt to solve everything in one go. The Jacobi Method works best when each equation in the system is diagonally dominant – a fancy way of saying that the value on the main diagonal (the position where the row number equals the column number) is larger than the other values in that row. When this condition is met, the Jacobi Method is likely to “converge,” or get closer to the correct answer over time.
Also Read: 12 Best Financial Engineering Programs in 2024
How the Jacobi Method Works
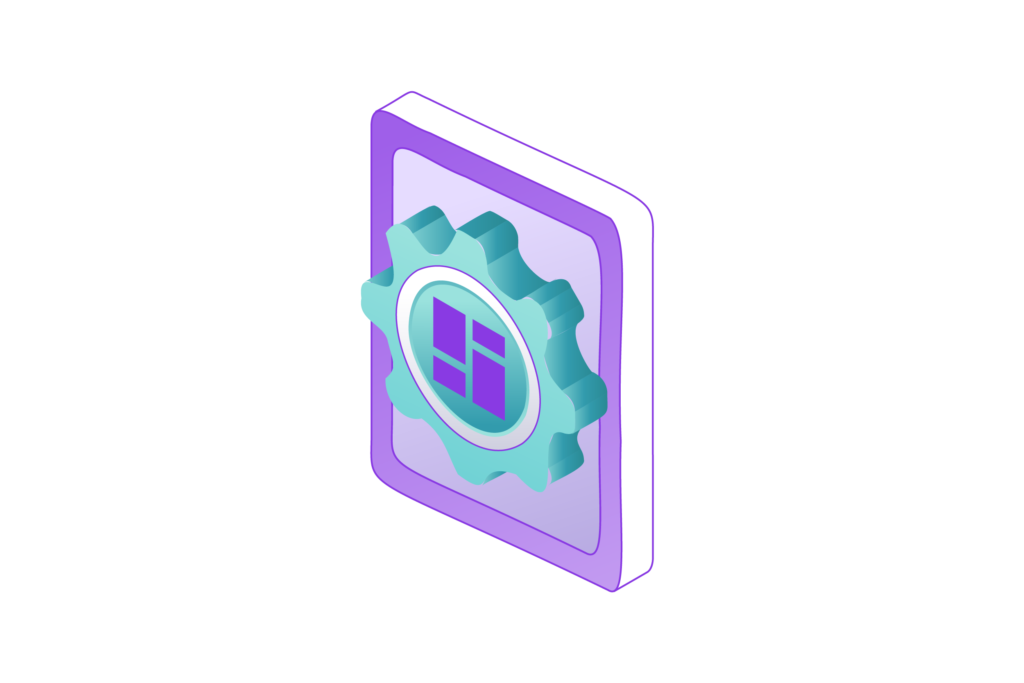
The Jacobi Method works by breaking down each equation and solving it independently, one variable at a time. It uses an initial guess for each unknown variable and refines this guess by repeatedly updating the values. Here’s a simple breakdown of how it goes:
- Start with a Guess: Begin by making an initial guess for each variable in the system. This can be any value, but a guess close to the actual solution can help the method reach the solution faster.
- Update Each Variable Independently: For each variable, rewrite the equation so that it’s isolated on one side. Then, plug in the current guesses for the other variables to calculate an updated value for that variable. The beauty of this method is that each variable can be updated separately, making it possible to calculate each one in parallel if you have multiple processors available.
- Repeat Until Convergence: Each time you go through all the equations and update the variables, you get a bit closer to the solution. This process, known as iteration, continues until the changes in values are so small that they’re within an acceptable range of error – at which point, we say the method has “converged.”
The Jacobi Method’s iterative nature is both its strength and limitation. It’s excellent for large problems where direct methods struggle, but it requires the system of equations to be well-behaved (diagonally dominant, ideally) for reliable results. In cases where this condition isn’t met, other methods like Gauss-Seidel might be more effective. However, when the setup is right, the Jacobi Method is a straightforward, powerful way to solve complex systems with Python.
Setting Up the Jacobi Method in Python
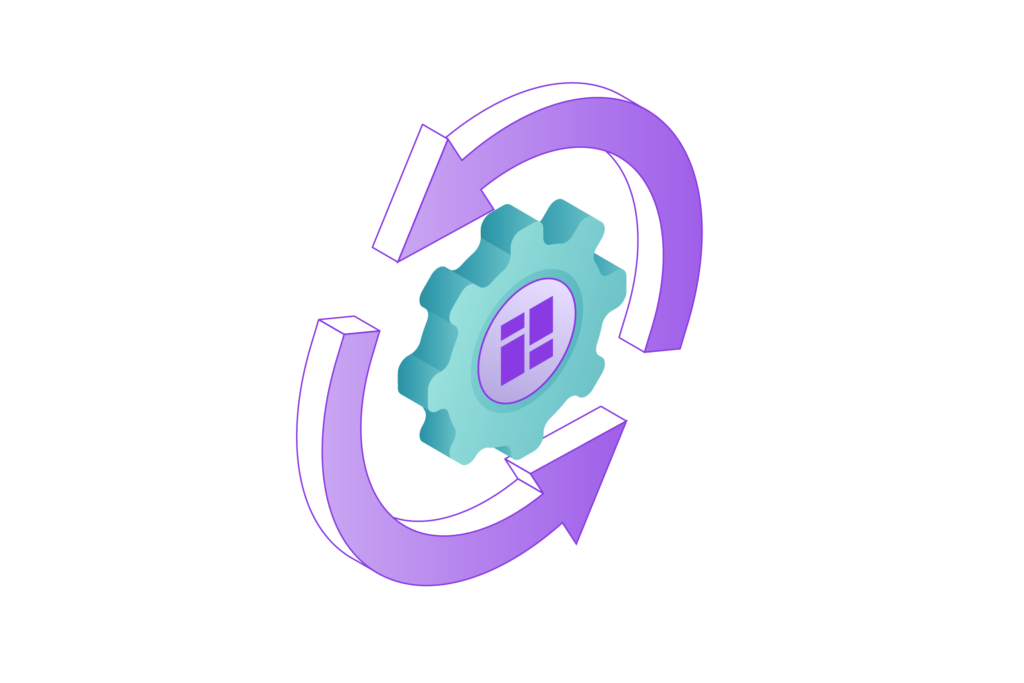
Now, let’s bring the Jacobi Method to life in Python! This setup involves a few essential tools, namely the NumPy library, which will help us handle matrix operations. If you haven’t used NumPy before, no worries – it’s a powerful library for scientific computing in Python, and it simplifies tasks like matrix calculations that are at the core of the Jacobi Method.
Step 1: Install NumPy
To get started, make sure you have NumPy installed. You can do this by running:
NumPy makes it easy to work with arrays, which will serve as the “containers” for our matrix and variables. The array format lets us perform operations across rows and columns in a way that’s both efficient and easy to read in code.
Step 2: Define the System of Equations
The Jacobi Method requires us to set up the system of linear equations as two main parts: the matrix of coefficients and a separate array for the results of each equation. For example, if we have three equations like this:
We can break it down as follows:
- Coefficient Matrix (A): Contains only the coefficients of each variable.
- Result Vector (B): Contains the constants from the right side of each equation.
Here’s how you’d set it up in Python:
Step 3: Set the Initial Guess
The Jacobi Method needs an initial guess to start the iteration. You can set this to zeros, or if you have an idea of the solution’s range, you can start with values close to it:
Now we’re ready to dive into the actual Jacobi Method implementation, where we’ll set up the loop to refine our guesses until we reach a solution!
Implementing the Jacobi Method in Python: A Step-by-Step Guide
With our system of equations and initial setup in place, it’s time to code the Jacobi Method in Python. This implementation will involve a loop that iterates until our solution reaches the desired level of accuracy, called “tolerance.” Let’s break down the code and understand each part.
Step 1: Set Up the Tolerance and Maximum Iterations
To determine when the solution is “close enough,” we’ll define a tolerance level – a small value that tells the loop to stop once the change in values becomes negligible. Additionally, we’ll set a limit on the number of iterations to avoid the program running indefinitely if convergence isn’t happening.
Step 2: Write the Jacobi Iteration Loop
Now we’ll set up the main loop that updates each variable one at a time based on the most recent values from the previous round. We’ll keep track of the difference between the new and old values to check if it’s within our tolerance.
Step 3: Understanding the Code
- Copy the Current Values: We start each iteration by copying the current values in x. This helps us calculate the new values (x_new) without accidentally changing the current values mid-calculation.
- Update Each Variable: For each variable iii, we calculate a sum that represents the influence of all other variables on the equation. This sum (sum_except_i) is subtracted from the constant term for that equation (B[i]), then divided by the coefficient of the current variable (A[i][i]) to get the new value for x[i].
- Check Convergence: The np.allclose function compares the old and new values of x to see if the difference between them is less than our tolerance. If yes, the loop breaks, signaling convergence.
- Max Iterations: If the solution doesn’t converge within the maximum iterations, the code ends and prints a message saying it didn’t converge.
Step 4: Print the Solution
Once the loop finishes, x holds our solution. You can print it to see the values of each variable:
And that’s it! You now have a working Python implementation of the Jacobi Method. Run this code to see how it iteratively hones in on a solution to the system of equations you set up.
Also Read: How to Succeed in a Quant Hedge Fund: Strategies and Tips
Advantages and Limitations of the Jacobi Method
Like any method, the Jacobi Method has its strong points as well as some limitations. Understanding these can help you decide when and where to use it effectively.
Advantages of the Jacobi Method
- Parallel Processing Potential: One of the Jacobi Method’s biggest strengths is that it updates each variable independently. This feature allows each equation to be solved separately, meaning it can be processed in parallel. For systems with many equations, parallel processing can speed up computations significantly, especially on modern computers with multiple processors.
- Simplicity and Ease of Implementation: The Jacobi Method is straightforward to code, as you’ve seen. Its structure is clear and doesn’t require complex calculations or additional storage beyond the main matrix and solution vectors. This simplicity makes it a good choice for learning about iterative methods and for use in situations where you need a quick solution without intensive setup.
- Flexibility for Large Sparse Systems: When solving large systems with many zero elements (sparse matrices), the Jacobi Method can be efficient. It avoids the direct manipulation of dense matrices, which can save both memory and computational resources, making it a practical choice for large-scale applications.
Limitations of the Jacobi Method
- Dependence on Diagonal Dominance: The Jacobi Method works best when the matrix of coefficients is diagonally dominant, meaning that the main diagonal values are larger than the sum of the other values in each row. When this condition isn’t met, the method may struggle to converge or may not converge at all. For non-diagonally dominant matrices, other methods like Gauss-Seidel are often more effective.
- Slow Convergence for Certain Systems: Compared to some other iterative methods, the Jacobi Method can take more iterations to reach an acceptable level of accuracy. While this isn’t a major problem for simple systems, it can be an issue when working with highly complex or sensitive systems. In such cases, alternative methods or even enhancements to the Jacobi Method, like preconditioning, may be necessary.
- Not Always Practical for High Accuracy: Since the Jacobi Method relies on approximations that improve over many iterations, it may not be ideal for applications where you need extremely high accuracy right away. For high-precision work, direct methods or faster-converging iterative methods may be preferable.
The Jacobi Method is a valuable tool, especially for specific types of problems. Understanding its strengths and limitations can help you decide when it’s the best fit and when to consider other approaches.
Conclusion
The Jacobi Method offers a practical and accessible approach to solving systems of linear equations, especially in Python, where tools like NumPy make matrix calculations simpler. By breaking down the problem into smaller, iterative steps, the Jacobi Method provides a way to tackle large or complex systems without overwhelming memory or processing power. If you’re a Python programmer or a student of numerical analysis, mastering this method can give you a valuable tool for handling linear systems.
One of the things that makes the Jacobi Method appealing is its suitability for parallel processing. In a world where computing power is often split across multiple processors, algorithms like Jacobi that can work on parts of the solution simultaneously are highly valuable. While it has its limitations – especially for non-diagonally dominant matrices or cases needing rapid convergence – the method remains relevant for a range of practical applications.
Whether you’re working on scientific computations, exploring mathematical modeling, or simply diving deeper into Python’s capabilities, experimenting with the Jacobi Method can deepen your understanding of iterative techniques. Try adjusting the parameters, experimenting with different initial guesses, or even comparing it to other methods to see what works best for your specific problem. As you gain more experience, you’ll find that iterative methods like Jacobi can open up a world of possibilities in computational math.
Disclaimer: The information provided by Quant Matter in this article is intended for general informational purposes and does not reflect the company’s opinion. It is not intended as investment advice or a recommendation. Readers are strongly advised to conduct their own thorough research and consult with a qualified financial advisor before making any financial decisions.
Joshua Soriano
As an author, I bring clarity to the complex intersections of technology and finance. My focus is on unraveling the complexities of using data science and machine learning in the cryptocurrency market, aiming to make the principles of quantitative trading understandable for everyone. Through my writing, I invite readers to explore how cutting-edge technology can be applied to make informed decisions in the fast-paced world of crypto trading, simplifying advanced concepts into engaging and accessible narratives.
-
Joshua Soriano#molongui-disabled-link
-
Joshua Soriano#molongui-disabled-link
-
Joshua Soriano#molongui-disabled-link
-
Joshua Soriano#molongui-disabled-link