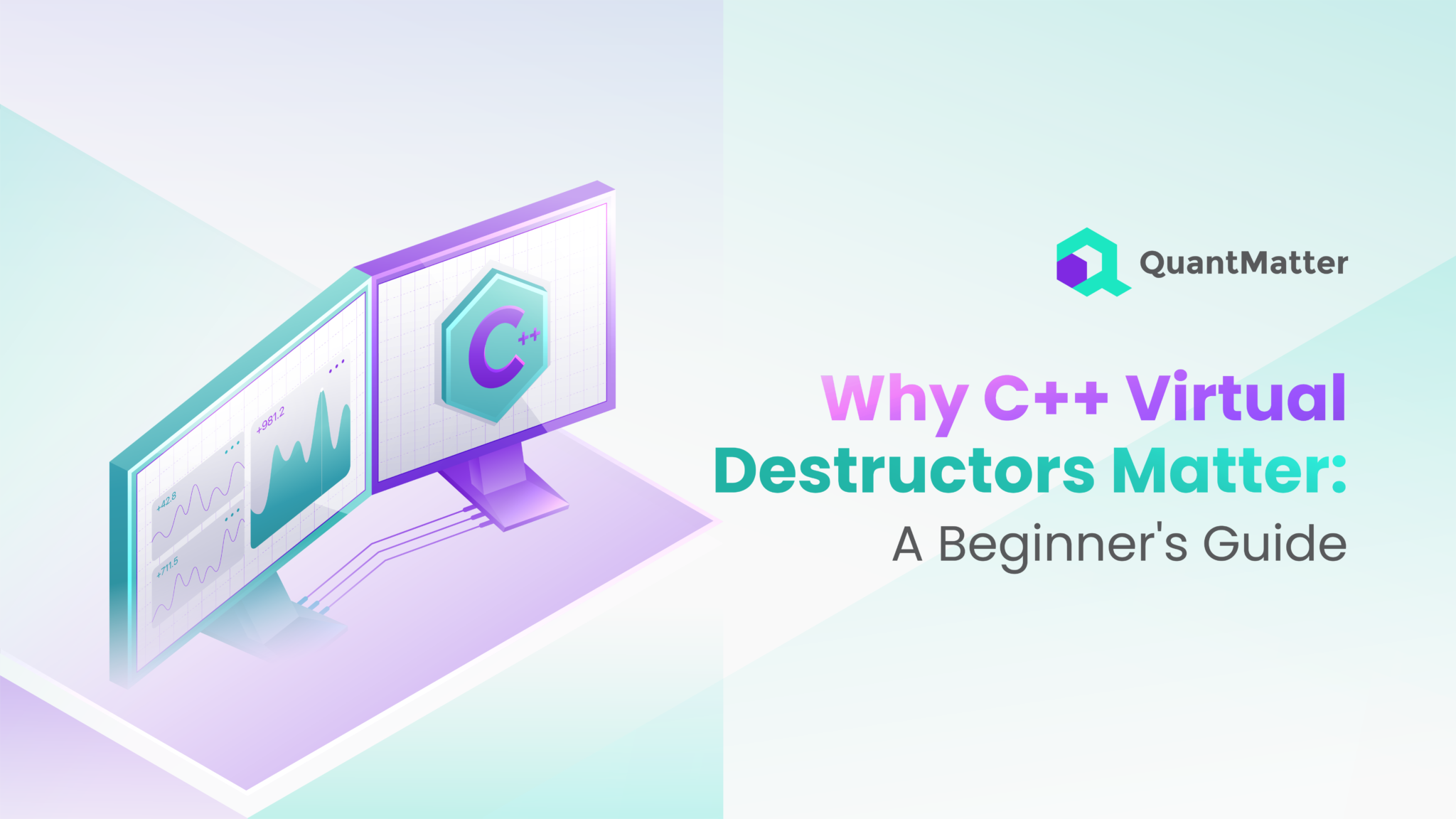
In this beginner’s guide, we will break down what destructors and virtual destructors are, why they matter in C++, and how to use them effectively to avoid common pitfalls. By the end, you’ll have a solid understanding of why virtual destructors are essential in C++ programming, especially when working with inheritance.
In C++, destructors are a critical part of managing an object’s lifecycle. They ensure that the resources allocated to an object are properly released when that object is no longer needed. However, when dealing with inheritance and polymorphism, a standard destructor might not behave as expected, which is where virtual destructors come into play.
Virtual destructors in C++ play a key role in ensuring that the correct destructor is called when an object is deleted through a pointer to a base class. This is particularly important in polymorphic classes, where objects of derived classes may be referred to using base class pointers.
What Are Destructors in C++?
In C++, a destructor is a special member function of a class that gets called automatically when an object of the class is destroyed. Destructors are responsible for freeing up resources, such as memory or file handles, that the object might have acquired during its lifetime. The main goal of a destructor is to ensure that when an object is no longer needed, it can clean up after itself to avoid resource leaks.
Destructors in C++ are denoted by a tilde (~) followed by the class name. For instance, if you have a class called MyClass, the destructor will be defined as ~MyClass(). The destructor is automatically invoked when the object goes out of scope or is explicitly deleted. Unlike constructors, a class can only have one destructor, and it doesn’t take any parameters or return any values.
A typical use case of destructors is in classes that manage dynamic memory. When a class allocates memory with new inside its constructor, the destructor is where delete is called to release that memory. Without a proper destructor, this allocated memory would remain occupied, leading to memory leaks.
Understanding Virtual Functions in C++
In C++, virtual functions are essential for enabling polymorphism, allowing objects of different derived classes to be treated as instances of a common base class. When a function in a base class is declared as virtual, it allows derived classes to provide their own implementation of that function. This is critical when working with inheritance.
The key benefit of virtual functions is that they enable dynamic dispatch, meaning the correct function is chosen at runtime based on the actual type of the object, rather than the type of the pointer or reference being used. Without virtual functions, the function call would be determined at compile time, which could lead to incorrect behavior in polymorphic systems.
Virtual functions allow developers to create flexible and reusable code by letting derived classes modify or extend the behavior of base class functions. This flexibility is especially useful when designing frameworks or libraries where the exact behavior of certain functions might change depending on the object being used.
Why Are Virtual Destructors Important?
Virtual destructors are a crucial feature in C++ that ensures proper memory management and resource cleanup, especially in applications that utilize polymorphism. When working with inheritance, it’s common to manipulate objects of derived classes through pointers to base classes. If a base class does not have a virtual destructor, deleting an object through a base class pointer can lead to significant issues like resource leaks and improper destruction of derived class members.
How Memory Leaks Occur Without Virtual Destructors
Memory leaks are one of the most common problems that arise when a destructor is not made virtual. If a base class destructor is not marked as virtual, deleting an object through a base class pointer will only invoke the destructor of the base class. This means that any dynamic memory or resources allocated by the derived class will not be released because the derived class destructor is never called. The result? The program continues running without freeing up memory, which can lead to resource exhaustion over time.
This kind of memory leak is particularly problematic in long-running applications, where unreleased resources can accumulate, leading to performance degradation or crashes. In more severe cases, these memory issues can be difficult to debug because they may not manifest immediately and might only appear under specific conditions, making them hard to detect and correct.
Ensuring Proper Resource Cleanup
The main reason for having a virtual destructor in the base class is to ensure that when a pointer to a base class deletes an object of a derived class, the destructor of the derived class gets called first, followed by the base class destructor. This cascading destructor call ensures that all the resources, including dynamically allocated memory, file handles, or other system resources managed by the derived class, are properly released before the base class destructor finishes the cleanup process.
In cases where the derived class allocates special resources or performs complex initialization in its constructor, it is even more important that these resources are released in the correct order. Virtual destructors guarantee that the cleanup happens systematically, with the most derived class destructed first, followed by the base class.
Avoiding Undefined Behavior
When a program fails to call the correct destructor for a derived class, it can result in undefined behavior, a term used in programming to describe operations that have unpredictable results. Undefined behavior might not just lead to memory leaks but also cause crashes or unexpected behavior in the program. This can make your code brittle, especially in larger, more complex systems where inheritance hierarchies are common.
For example, in the absence of a virtual destructor, pointers to base classes might not free up resources as expected. This can cause your program to become unreliable, behaving differently on different machines or under different conditions. By adding a virtual destructor, you mitigate the risk of these hard-to-diagnose issues, ensuring that objects are destroyed correctly and consistently.
Best Practices for Using Virtual Destructors
Any time you design a class to be used as a base class in a polymorphic hierarchy, it’s considered a best practice to declare the destructor as virtual. This doesn’t incur a significant performance penalty in most cases, but it adds a layer of safety that ensures objects are cleaned up properly. It’s particularly important in projects that are expected to evolve over time, where new derived classes might be added later.
Even if a base class doesn’t currently manage any dynamic resources, making its destructor virtual is a good habit to develop, as this ensures that future developers (or even you) won’t have to worry about adding it later when the class hierarchy becomes more complex.
How to Implement a Virtual Destructor in C++
Implementing a virtual destructor in C++ is a simple yet important step in managing memory when working with inheritance. While the implementation itself is straightforward, its impact on the behavior of your class hierarchy is significant, particularly when dealing with polymorphism.
Declaring a Virtual Destructor
To make a destructor virtual, you only need to use the virtual keyword when declaring the destructor in the base class. This tells the compiler to use dynamic dispatch for the destructor, ensuring that the appropriate destructor in the inheritance hierarchy is called.
Once the destructor is marked as virtual in the base class, any derived class will automatically have its destructor called when an object is destroyed, even if it’s being referenced by a base class pointer.
Why Only the Base Class Needs a Virtual Destructor
It’s important to note that you only need to declare the destructor as virtual in the base class. The derived classes inherit this behavior automatically. Once the base class destructor is marked as virtual, the destructors of all derived classes are also treated as virtual, even if you don’t explicitly declare them that way.
This simplifies the management of large class hierarchies, as you don’t need to mark every destructor in the hierarchy as virtual. Just ensuring that the base class has a virtual destructor is enough to guarantee correct behavior.
Performance Considerations of Virtual Destructors
While virtual destructors add a small overhead due to the use of a virtual table (vtable), this overhead is minimal in most real-world applications. The slight performance cost of a virtual destructor is generally outweighed by the benefits of correct and predictable resource management, especially when working with dynamic memory or complex class hierarchies.
In most cases, avoiding memory leaks, undefined behavior, and other issues related to improper destruction far outweighs the marginal cost of making a destructor virtual. For the majority of applications, this overhead is not noticeable and should not be a concern.
How Virtual Destructors Work in Complex Inheritance Chains
In complex inheritance hierarchies, where multiple layers of derived classes exist, the virtual destructor ensures that destructors are called in the correct order. When an object of the most derived class is deleted through a pointer to a base class, the destructor of the most derived class is invoked first. Then, destructors of intermediate classes are called, and finally, the base class destructor is executed.
This orderly destruction process ensures that resources are released systematically. It also protects against potential issues that could arise if the derived class destructor was skipped, such as failure to release memory or close file handles.
Virtual Destructors in Abstract Base Classes
Virtual destructors are particularly important in abstract base classes (or pure virtual classes), where the class is intended to serve only as a base for other derived classes. Since these base classes are never instantiated directly, but through derived classes, having a virtual destructor ensures that when a derived object is deleted, its destructor and any necessary cleanup operations are performed correctly.
Common Mistakes When Using Virtual Destructors in C++
Even though virtual destructors are a critical part of memory management in C++ polymorphism, it’s easy to overlook them or use them incorrectly, especially for beginners. Understanding the common mistakes when working with virtual destructors can help you avoid pitfalls that might lead to resource leaks, undefined behavior, or inefficiencies in your code.
Forgetting to Make the Destructor Virtual
One of the most frequent mistakes is forgetting to declare the destructor in a base class as virtual. When a base class destructor is not virtual, deleting a derived class object through a pointer to the base class will only call the base class destructor, ignoring any cleanup that the derived class might need to perform. This is a serious problem in polymorphic systems where derived classes manage resources, such as dynamic memory.
In complex systems, this mistake can lead to subtle bugs that may not be immediately apparent. Over time, these issues can degrade the performance of an application, leading to memory leaks and other resource management problems.
Adding a Virtual Destructor When It’s Unnecessary
While virtual destructors are important in polymorphic class hierarchies, they aren’t needed for every class. If a class is not meant to be used as a base class for polymorphism, marking the destructor as virtual adds unnecessary overhead. Non-polymorphic classes don’t need virtual destructors because they are never deleted through pointers to base classes.
Adding a virtual destructor to a class that doesn’t need it introduces the overhead of maintaining a virtual table (vtable) for that class. While this overhead is generally small, it’s best practice to avoid it unless necessary. Only make the destructor virtual if you intend for the class to be a base class in a polymorphic hierarchy.
Not Implementing a Destructor in Derived Classes
Another common mistake is failing to implement a destructor in derived classes when one is needed. Even if the base class destructor is correctly marked as virtual, derived classes that manage their own resources should also have explicit destructors. If a derived class allocates memory or acquires resources in its constructor, it needs a destructor to properly release those resources.
Without a destructor in the derived class, memory or resource leaks can still occur, even if the base class destructor is virtual. Always ensure that derived classes have destructors when they need to manage their own resources, such as dynamically allocated memory or file handles.
Overcomplicating Destructor Logic
Another pitfall that developers may fall into is making destructors overly complicated. While it’s tempting to put complex cleanup logic into destructors, it’s generally a good idea to keep them as simple as possible. Destructors should focus solely on releasing resources like memory, file handles, or database connections. Complex logic in destructors can make them harder to maintain and debug, increasing the risk of errors.
Additionally, destructors should not throw exceptions. Throwing exceptions from a destructor can lead to program termination if another exception is already being handled. This makes exception handling more difficult and can result in unexpected program behavior. Always keep destructor logic simple and avoid any operations that could fail or throw an exception.
Using Virtual Destructors in Performance-Critical Code
While the overhead of a virtual destructor is generally negligible, there are cases where it might affect performance, particularly in performance-critical code such as real-time systems or low-latency applications. In these scenarios, the use of virtual destructors might introduce overhead that can be avoided by designing the class hierarchy differently.
In such cases, it’s important to profile the code and determine whether the virtual destructor is truly necessary or if the class hierarchy can be restructured to avoid the need for polymorphism in performance-critical sections of code.
Conclusion
Understanding virtual destructors in C++ is crucial for anyone working with polymorphism and dynamic memory allocation. By ensuring that the correct destructors are called, you prevent memory leaks, resource mismanagement, and undefined behavior. Failing to implement virtual destructors in a base class can lead to subtle but significant bugs that can be difficult to track down later.
For beginners, it might seem like an advanced concept, but with practice, you’ll see how virtual destructors are just another tool in the C++ toolbox for effective memory management. Using virtual destructors allows you to work with complex object hierarchies confidently, knowing that the destructors will behave as expected.
As you continue your journey with C++, understanding and implementing concepts like virtual destructors will become second nature. They are not just a technical detail, but a fundamental part of writing robust, maintainable, and efficient C++ code.
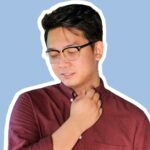
Joshua Soriano
As an author, I bring clarity to the complex intersections of technology and finance. My focus is on unraveling the complexities of using data science and machine learning in the cryptocurrency market, aiming to make the principles of quantitative trading understandable for everyone. Through my writing, I invite readers to explore how cutting-edge technology can be applied to make informed decisions in the fast-paced world of crypto trading, simplifying advanced concepts into engaging and accessible narratives.
- Joshua Soriano#molongui-disabled-link
- Joshua Soriano#molongui-disabled-link
- Joshua Soriano#molongui-disabled-link
- Joshua Soriano#molongui-disabled-link